

 |
Search |
 |
|

 |
Classic 2 Guys |
 |
10 Random Stories:





|
|
 |

 |
A PHP Tutorial - Part 1 |
 |
This is a tutorial to help you get started with PHP. If you don't know what PHP is or why you would use it, check out my previous article, PHP - The Very Basics.
I'm going to assume you have PHP installed, Web Sharing enabled, and that you have at least a little bit of experience with some other scripting or programming language (but don't worry, we'll take it slow). So get strapped in, put your cheese helmet on, and let's get PHP'in!
Make your first PHP script
Open up a text editor and enter the following code:
<?php phpinfo(); ?>
Save the file as "test.php" where you have your shared Web documents (On OS X this is "Library/WebServer/Documents/"). Now launch a Web browser and go to "localhost/test.php".
If successful, you'll see a huge list that tells you all about your PHP installation. Don't worry if it doesn't make sense, it shouldn't at this point. You should be proud, you wrote your first PHP script! Print it out and stick it on the fridge.
Now to explain what you typed in, you have to understand that PHP and HTML (and just plain text) can be used in the same PHP file. The PHP engine will only parse chunks of text that you designate as PHP code.
More simply put, this starts PHP code:
<?php
or just:
<?
And this ends PHP code:
?>
phpinfo() is just a call to one of PHP's many built-in PHP functions. Finally, statements in PHP are terminated with a semicolon.
The Echo command
Now let's get into stuff that will actually let you do stuff...and stuff.
To print something out to the browser, you do an echo command, like so:
<?
echo ("You are a crazy PHP learnin' fool!");
?>
Anything in between the quotes is printed on the page. Try it out on a new PHP file. Neat, huh? It gets much better!
Variables
I hope you know what variables are because explaining them is beyond the scope of this tutorial. (Although if you email me or leave a comment I'd be happy to point you in the right direction.)
A variable in PHP is easily declared like so:
$someNumber = 10;
We're creating a variable name "someNumber" and assigning the value "10" to it. The above statement reads "someNumber gets 10".
You can also assign text to a variable:
$someText = "I like to dance around in a frilly tutu.";
You could then echo that variable out to the browser:
echo ($someText);
This would tell the world you like to dance around in a tutu. Maybe not something you'd like to share, but that's up to you.
Operations on Variables
You can do all the usual math operations you'd expect with variables. Let me introduce comments for these examples. Single-line comments are started with a double-slash "//".
$x = 10; // $x gets assigned the number 10
$y = $x + 5; // $y gets 10 + 5, or 15
echo $y; // "15" will be printed to the screen.
Note that we didn't use parenthesis around what we echoed - you can take them or leave them, they're purely for readability's sake. I use them to surround text, but not around variables.
Likewise you can add, subtract or multiply (or even modulus) variables. Some examples:
$x = 10;
$x = $x - 5; // $x is now equal to 5.
$x = $x * 2; // Asterisk is the multiplication symbol. $x is equal to 10.
$x = $x / 2; // We're doing division. $x equals 5.
Text Manipulation
Now for string variables. We can concatenate variables containing text like so:
$first = ("Don't slap the pig. ");
$second = ("Slap the monkey.");
$full = $first.$second;
echo $full; // The result is "Don't slap the pig. Slap the monkey."
Combining strings like this can be really useful. To look at a real-world example, let's make templates for a pretend web site.
If you've ever made your own Web site containing multiple pages, you've probably found that it's tedious to change information, such as navigation links or other header information, for each and every page in your site.
Well, you could have variables containing the text for your header or footer files like so:
$header = "Welcome to my site!";
$footer = "Site made by me.";
$pageText = $header."
Here's the body of the page.
".$footer;
Now if we echoed $pagetext out to the browser we'd have:
Welcome to my site!
Here's the body of the page.
Site made by me.
Now that's really not doing a whole lot, as you would have to specify the $header and $footer variables on each every page. The way to really do this would be to define a function, or chunk of code, to be used over and over by many different files.
But that'll have to wait until the next article, unfortunately. We'll also cover 'include' files, arrays, and more. As always, let me know if you have any questions!
|
|
May 29 2003, 8:02 AM EDT, by
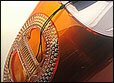 |
Comments:
|
Randeep Nanák |
5/29/03, 4:27 PM EDT |
Hi Jonathan,
Do you have a book on PHP or would you like to recommend one so that I could use and learn.
[email protected]
|
Jonahan |
5/29/03, 4:41 PM EDT |
Randeep, I'd recommend HP and MySQL Web Development. It's in it's second edition and I personally think it's a fantastic book.
It starts out at a very beginner level, and eases you along.
And oh yeah, I did mention it in my previous article ( I just read Jedbeck's post), but that's ok :)
I can't really recommend anything else, because that was the only book I needed. I also frequented www.php.net to supplement my learning.
|
stickman67 |
5/29/03, 7:34 PM EDT |
Has anyone seen my cheese helmet? Damn! No, the salami one is no good at all! Now I'll never be able to code in PHP! *Sob!*
|
Jonahan |
5/30/03, 1:50 PM EDT |
Um...sorry...I ate it.
I'll make you another one though - you'll need it to deflect the Super Stupidity Rays coming from Microsoft's "Make Everyone Stupid So They'll Buy Our Stuff" tower in Redmond.
Is Limberger alright?
|
stickman67 |
5/30/03, 9:07 PM EDT |
Limberger's good, though I've always had a hankering for a blue-vein helmet ... Oh no! What am I saying? Have I just "come out" of the refrigerator? Noooooo!
|
Jonahan |
6/1/03, 3:37 PM EDT |
Haha ;)
I'd never heard of blue-vein cheese...ya learn something new every day!
|
This article is archived, so you may not comment on it.
(The good news is there's always the shoutbox, the forums or the contact form if you're socially-inclined at the moment!)
|
|
 |
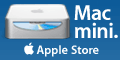

 |
Site Links |
 |
|
 |
Deep Thoughts |
 |
When you die, if you get a choice between going to regular heaven or pie heaven, choose pie heaven. It might be a trick, but if it's not, mmmmmmm, boy.
|
 |
Around Da Web |
 |
iProng: |
iPhone steals show at CTIA Wireless 2007
|
DLO offers dual cover fashion case for iPod
|
AT&T received 1M inquiries on iPhone
|
MacDailyNews: |
Ars Technica in-depth review: Apple TV ?impressed all those who touched it?
|
Inside Apple?s Mac OS X 10.5 Leopard Server OS
|
The chips inside Apple TV
|
Think Secret: |
Adobe Creative Suite 3 pricing revealed
|
|
 |
We Like: |
 |
|
 |
Side Projects |
 |
Jonahan
- JediPoker.net
- Jonahan.com
- iProng
- MacProng
iKen
Jedbeck
J.P.
|
|